Fast Chebyshev Transform
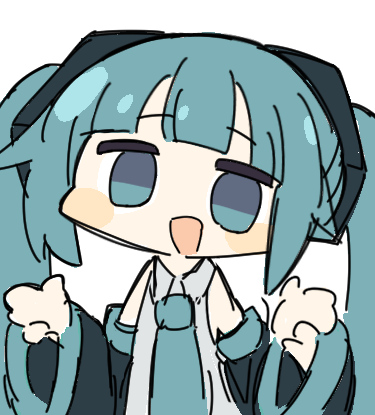
FFT & DCT
In MATLAB, the function fft
used to compute the Fast Fourier Transform (FFT) of a sequence of data points is defined as follows: Y = fft(X)
is the discrete Fourier transform (DFT) of vector
And the inverse FFT is given by X = ifft(Y)
, where
For Discrete Cosine Transform (DCT), which is closely related to the Chebyshev transform and discrete Fourier transform, MATLAB provides the functions dct
and idct
to compute the forward and backward DCT, respectively. The DCT has four standard variants. For a signal
- DCT-1:
- DCT-2:
- DCT-3:
- DCT-4:
The series are indexed from
All variants of the DCT are unitary (or, equivalently, orthogonal): To find their inverses, switch
Chebyshev Transform
Using the values of a function
we can define a discrete Chebyshev transform
These values
Using the discrete orthogonality of Chebyshev polynomials, namely
we can define the inverse Chebyshev transform as
where
which is symmetric in
Remark. It’s possible to define other forms of discrete Chebyshev transform based on any of the other orthogonality relations of Chebyshev polynomials.
Connection with FFT and DCT
The discrete Chebyshev transform defined above is intimately connected with the FFT and DCT. Assume
which are zeros of
then
for
for
for
for
for
for
In this manner, the Chebyshev transform can be computed via the FFT in
Numerical Implementation
Now we give a simple numerical example to illustrate the implementation of the Chebyshev transform via FFT, by using
1 | f = @(x) x.^2 + exp(x); % Test function |
This code uses the FFT to compute the forward and backward Chebyshev transform and compares the results with the Chebfun
package. The output of the code is
1 | ans = |
The results show that the Chebyshev transform computed by the FFT is consistent with the output given by Chebfun package, which is based on the Chebyshev interplation.
This method is simple but not optimal. We summarize this method in the following MATLAB code.
1 | % CHEBTRANSF Forward Chebyshev Transformation via FFT. |
Remark. To use the function ifft
by equation gk = [fk; fk(end-1:-1:2)]
to make the function even and periodic. Also, sometimes the sort of CGL points may be different from the standard one, for example, if using
In fact, the Chebyshev differentiation can also be computed via FFT. The following MATLAB code gives a simple implementation of the Chebyshev differentiation, which comes from [4].
1 | % CHEBFFT Chebyshev differentiation via FFT. Simple, not optimal. |
Reference
- Mathworks, Fast Fourier Transform
- Mathworks, Discrete Cosine Transform
- J.C. Mason, D.C. Handscomb, Chebyshev Polynomials, Chapman & Hall/CRC, 2003.
- L. N. Trefethen, Spectral Methods in Matlab, SIAM, 2000.
- Title: Fast Chebyshev Transform
- Author: Gypsophila
- Created at : 2025-03-26 15:14:27
- Updated at : 2025-03-26 21:55:48
- Link: https://chenx.space/2025/03/26/FastChebTransform/
- License: This work is licensed under CC BY-NC-SA 4.0.